What is Powershell?
PowerShell is a cross-platform automation and configuration management framework from Microsoft. It consists of a command-line shell, a scripting language, and a set of tools designed to automate administrative tasks, manage systems, and perform complex configurations.
Initially developed for Windows, PowerShell is now cross-platform, available on Windows, macOS, and Linux.
The Powershell Study Notes and Guide
Powershell Study Notes and Guide is a comprehensive guide for mastering PowerShell, covering everything from basic commands and syntax to advanced features like remote scripting, automation, system management, and cybersecurity. It’s tailored for IT professionals, sysadmins, and cybersecurity practitioners seeking practical knowledge and examples.
PowerShell is a powerful automation and scripting tool that blends a robust command-line interface with scripting capabilities. This guide offers exhaustive coverage of PowerShell fundamentals, including cmdlet syntax, pipelines, variables, data types, and providers. It delves into administrative tasks like system and user management, networking, scheduled tasks, and file manipulation.
Advanced topics such as PowerShell remoting, error handling, configuration management (including Desired State Configuration), and background jobs are treated with practical examples.
It concludes with cybersecurity applications like using PowerSploit and Nishang, emphasizing PowerShell’s relevance in red teaming and system defense. Whether you’re configuring servers or investigating malicious activity, PowerShell proves indispensable.
Table of Contents:
Setting up Powershell
Basics of Cmdlets and modules
Basic Syntax: Verb-Noun
Data Types in Powershell
Common Data Types in PowerShell
Variables in Powershell
Pipes
File System Navigation
Working with Date and Time
Filtering and Sorting
Interacting with The Web
System Management
Powershell Remoting
PS Providers
PSDrives in Powershell
WMI and CIM in PowerShell
Error Handling in Powershell
Configuration Management with Powershell
Background Jobs in PowerShell
Scripting
PowerShell Integrated Scripting Environment (ISE)
Basics of Writing Powershell Scripts
Loops
Conditions in Powershell
Comparison Operators in Conditions
Logical Operators
Scripting Constructs in PowerShell
Provisioning a New Server Core Instance with PowerShell
Example Scripts for IT Professionals
Example Scripts for Cyber Security
Common Powershell Tools in Cyber Security
Page Count: 232
Format: PDF
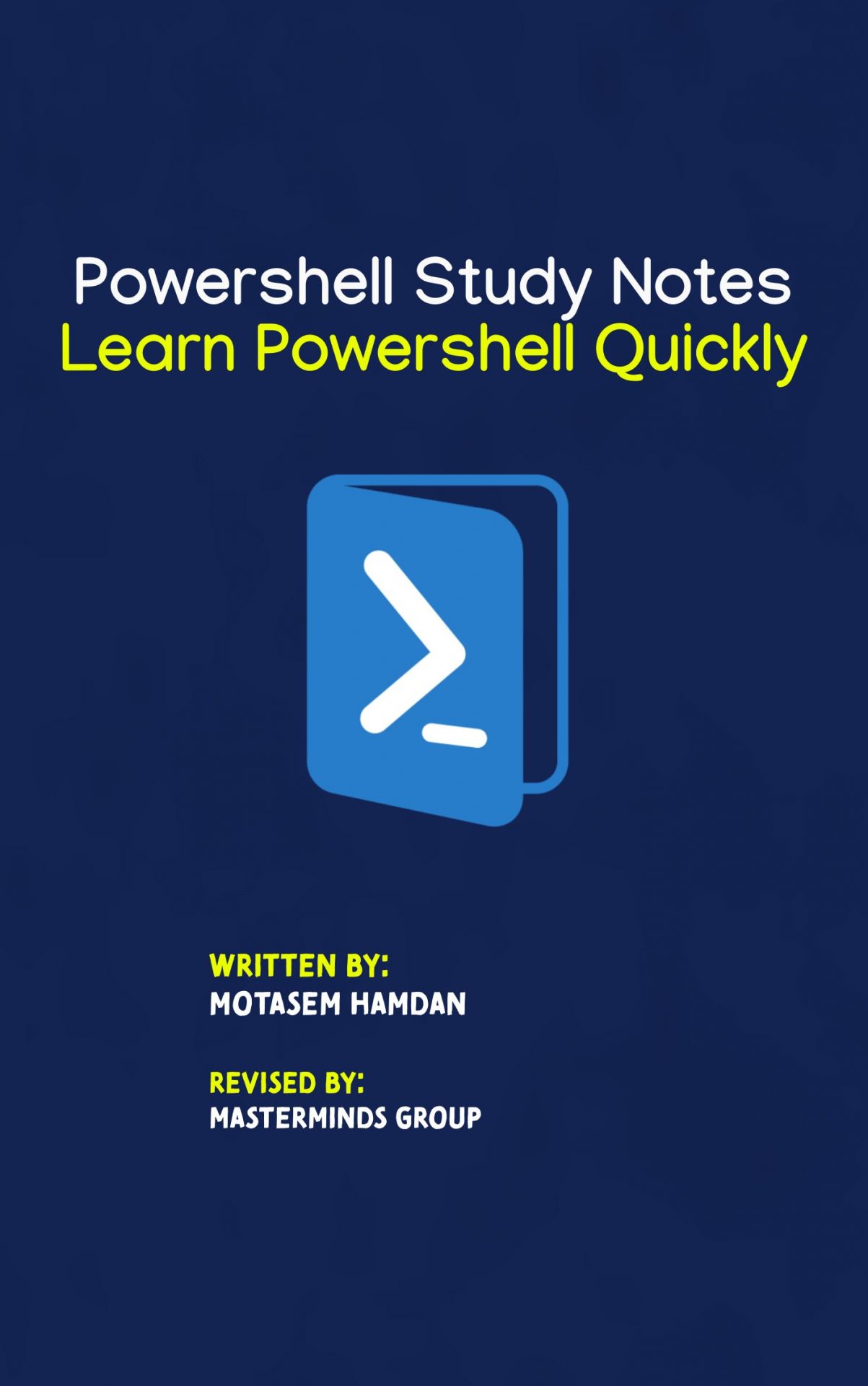
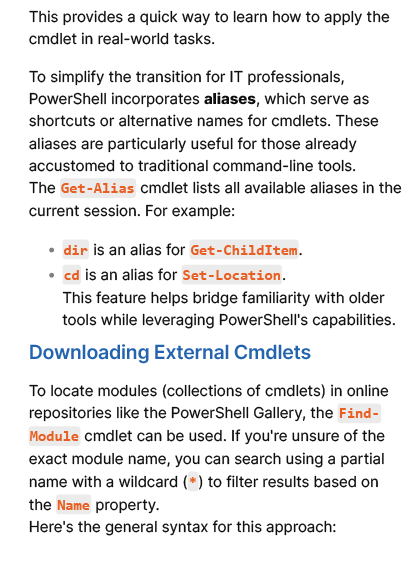
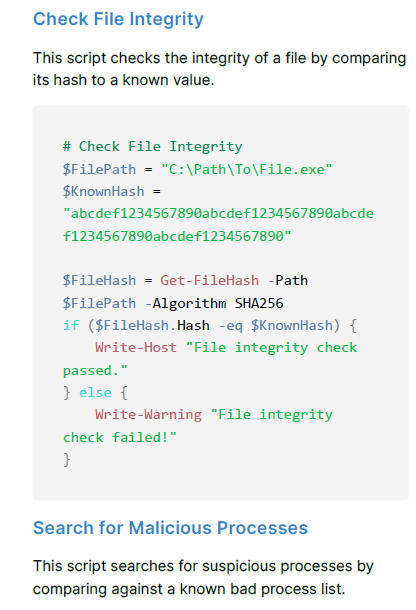
Testimonials (LinkedIn)
How to buy the E-book?
You can buy the book directly by clicking on the button below
After you buy the booklet, you will be able to download the PDF book.
Key Components of PowerShell
- Command-Line Shell:
- An interface to interact with the operating system and applications through commands.
- Scripting Language:
- A robust, object-oriented language designed for automating tasks and writing scripts.
- Modules and Cmdlets:
- Cmdlets (pronounced “command-lets”) are specialized commands in PowerShell.
- Modules are collections of related cmdlets, functions, and resources.
- Pipeline:
- PowerShell uses a pipeline (
|
) to pass the output of one command as input to another.
- PowerShell uses a pipeline (
PowerShell Features
1. Object-Oriented Design
- Unlike traditional shells (e.g., Command Prompt or Bash), PowerShell outputs objects instead of plain text.
- This allows commands to work seamlessly with structured data.
Example:
Get-Process | Select-Object Name, CPU
2. Cmdlets
- Small, single-function commands built into PowerShell.
- They follow the
Verb-Noun
naming convention (e.g.,Get-Process
,Set-Content
).
Example:
Get-Help Get-Process
3. Cross-Platform
- PowerShell Core (from version 6 onwards) runs on Windows, Linux, and macOS, making it suitable for managing diverse environments.
4. Scripting and Automation
- PowerShell scripts (saved as
.ps1
files) can automate repetitive tasks, manage system configurations, and handle bulk operations.
Example Script:
# Save to MyScript.ps1
Write-Host "Starting Process"
Get-Service | Where-Object { $_.Status -eq "Running" } | Select-Object Name, Status
Write-Host "Process Completed"
5. Remoting and Management
- PowerShell supports remoting to execute commands on remote systems using protocols like WSMan or SSH.
Example:
# Enable remoting
Enable-PSRemoting -Force
# Run command on a remote machine
Invoke-Command -ComputerName "Server01" -ScriptBlock { Get-Service }
6. Modules
- Extend PowerShell’s functionality with modules, which contain additional cmdlets, scripts, and resources.
- Popular Modules:
- Active Directory: For managing AD environments.
- Azure: For managing Microsoft Azure resources.
- PSWindowsUpdate: For managing Windows updates.
Example: Import a Module
Import-Module ActiveDirectory
Get-ADUser -Filter *
7. Integrated Scripting Environment (ISE)
- PowerShell ISE provides a graphical user interface for script editing, debugging, and testing.
Common Use Cases for PowerShell
- System Administration:
- Manage users, groups, and systems in Active Directory.
- Configure networking, services, and scheduled tasks.
- Automation:
- Automate repetitive tasks like software deployment, backups, and log analysis.
- Cloud and DevOps:
- Manage cloud platforms like Azure, AWS, or Google Cloud using dedicated modules.
- Automate CI/CD pipelines with tools like Azure DevOps.
- Security and Compliance:
- Audit system configurations, check for vulnerabilities, and enforce compliance policies.
- Scripting for Developers:
- Perform bulk operations, generate reports, or test environments programmatically.
PowerShell Versions
- Windows PowerShell:
- Default version in Windows environments up to version 5.1.
- Limited to Windows-only.
- PowerShell Core:
- Cross-platform from version 6.0.
- Built on .NET Core.
- PowerShell (7.x):
- The latest cross-platform version.
- Combines the features of Windows PowerShell and PowerShell Core.
Check Version:
$PSVersionTable
PowerShell Cmdlet Examples
- Retrieve System Information
Get-ComputerInfo
List Running Processes
Get-Process
Filter and Sort Files
Get-ChildItem -Path C:\ -Recurse | Where-Object { $_.Extension -eq ".txt" } | Sort-Object Length
Export Data to a CSV
Get-Service | Export-Csv -Path "C:\Services.csv" -NoTypeInformation
Query Active Directory
Import-Module ActiveDirectory
Get-ADUser -Filter * | Select-Object Name, Department
Advantages of PowerShell
- Unified Management:
- Manage local, remote, and cloud systems with a single tool.
- Extensibility:
- Add functionality using modules or custom scripts.
- Ease of Use:
- Intuitive cmdlets and syntax make it accessible for beginners.
- Cross-Platform Support:
- PowerShell Core/7.x enables seamless management across Windows, Linux, and macOS.
- Automation Capabilities:
- Simplify complex or repetitive administrative tasks.
PowerShell Learning Resources
- Built-In Help:
Get-Help <Cmdlet-Name>
Microsoft Docs:
- Official documentation and tutorials for PowerShell.
Community Resources:
- GitHub repositories and PowerShell blogs.
Practice Scripts:
- Start by automating small tasks like file management or system monitoring.
What about the notes updates?
if you have been following my YouTube Channel, you definitely know that those who subscribe to the second tier of my channel membership they instantly get access to a vast catalog of cybersecurity, penetration testing, digital marketing, system administration and data analytics notes catalog for 10$ along with the ability to receive all notes updates as long as they are subscribed so what does that mean?
This means if you want to stay up to date with the changes and updates to the notes and get access to other categories, I encourage to join the channel membership second tier instead. However, if you are fine with downloading the current version of this section of the notes then you can buy this booklet instead for a one-time payment.
Will the prices of this booklet change in the future?
Once another version of this E-book is released, which it will, the price will slightly change as the booklet will include more contents, notes and illustrations.
Powershell Basics for IT and Cybersecurity
Checkout the video below on my YouTube channel for free Powershell training