Introduction to Assembly Short Mini Course
In this short mini Assembly programming language course, we first go over the CPU architecture explaining different parts of the CPU and how it interacts with the RAM & and the input/output devices. We then pivot into discussing the different types of registers such as general purpose registers and the intruction pointer. Moving along, we cover the different sections of the memory including the data and code segment, in addition to the heap and the stack as well as the key differences between them.
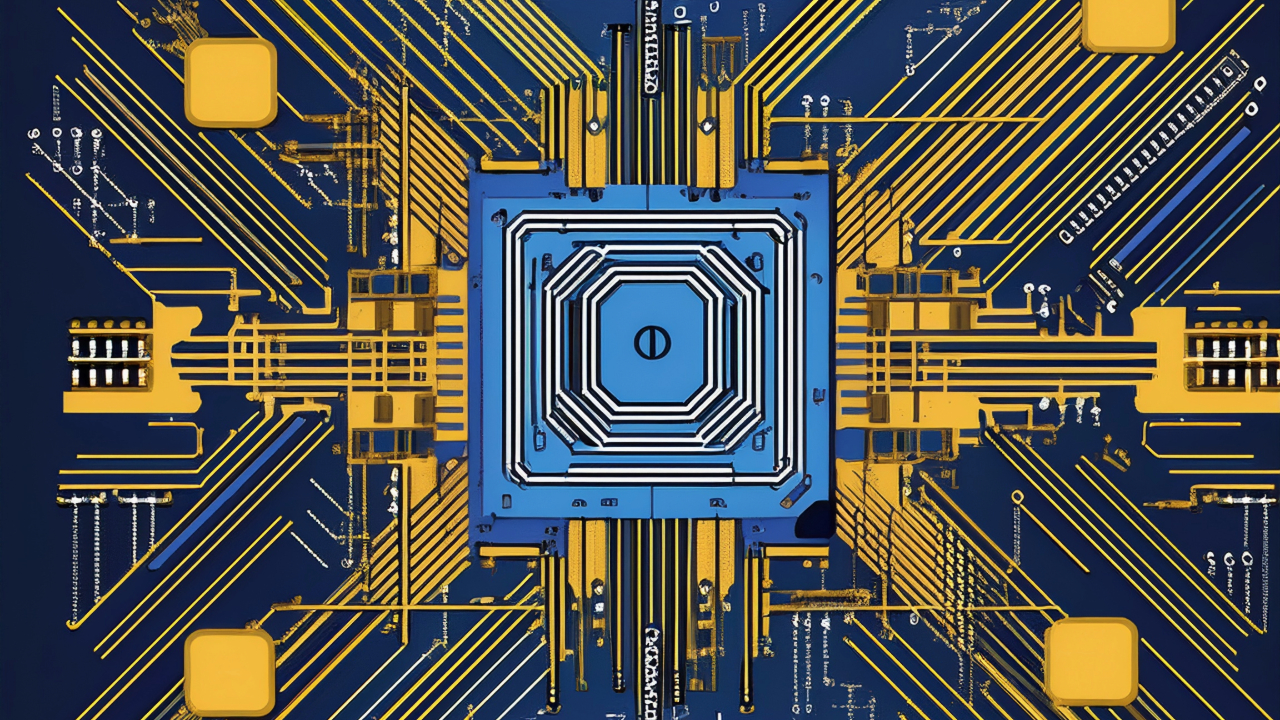
After briefly going over the x86 architecture, we explain the opcodes, operands, assembly insutrctions such as mov, push, pop, shift, and others. Lastly, we simulate an Assembly code execution flow while examining how the stack, registers and the memory change during every step. The simulation part is part of TryHackMe x86 Architecture Overview & x86 Assembly Crash Course rooms respecetively
Please watch the video at the bottom for full detailed explanation of the walkthrough.
Course Contents
- Understanding CPU Architecture
- Understanding Registers
- Understanding Memory (RAM)
- Stack vs Heap in Assembly
- Operands & Opcodes
- Assembly Instructions
- Practical Code Simulation
Overview of Assembly Programming Language
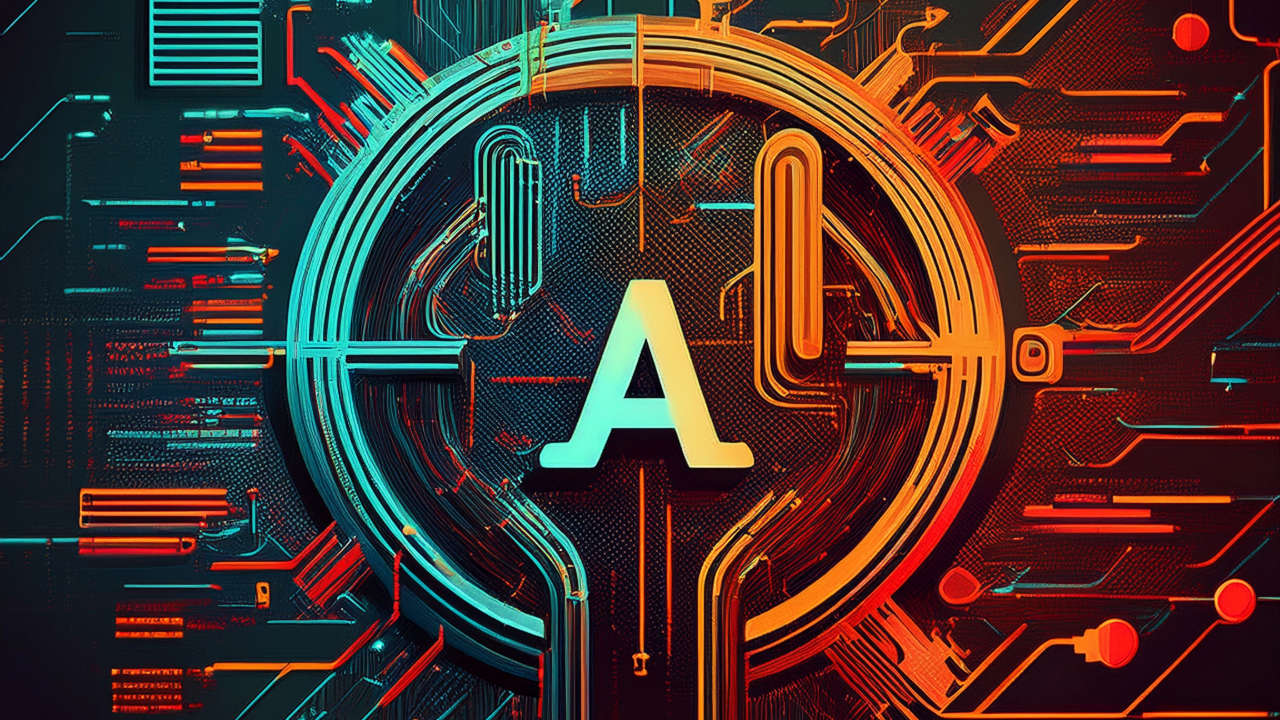
When learning malware reverse engineering, knowing the basics of assembly language is essential. This is because when we get a malware sample to analyze, it is most likely a compiled binary. We cannot view this binary’s C/C++ or other language code because that is not available to us. What we can do, however, is to decompile the code using a de-compiler or a disassembler. The problem with decompiling is that a lot of information in the written code is removed while it is compiled into a binary; hence we won’t see variable names, function names, etc., as we do while writing code. So the most reliable code we have for a compiled binary is its assembly code.
CPU Components
CPU consists of the following:
- Arithmetic Logic Unit (ALU): The arithmetic logic unit executes the instruction fetched from the Memory. The results of the executed instruction are then stored in either the Registers or the Memory.
- The Control Unit: The Control Unit gets instructions from the main memory (RAM). The address to the next instruction to execute is stored in a register called the
Instruction Pointer or IP
. In 32-bit systems, this register is calledEIP
, and in 64-bit systems, it is calledRIP
. - The Registers: The Registers are the CPU’s storage. Registers are generally much smaller than the Main Memory, which is outside the CPU, and help save time in executing instructions by placing important data in direct access to the CPU.
Memory (RAM)
The Memory, also called Main Memory or Random Access Memory (RAM), contains all the code and data for a program to run. When a user executes a program, its code and data are loaded into the Memory, from where the CPU accesses it one instruction at a time.
Recommended Resources
TryHackMe x86 Architecture Overview
TryHackMe x86 Assembly Crash Course
Room Answers | TryHackMe x86 Architecture Overview
In which part of the Von Neumann architecture are the code and data required for a program to run stored?
Memory
What part of the CPU stores small amounts of data?
Registers
In which unit are arithmetic operations performed?
Arithmetic Logic Unit
Which register holds the address to the next instruction that is to be executed?
Instruction Pointer
Which register in a 32-bit system is also called the Counter Register?
ECX
Which registers from the ones discussed above are not present in a 32-bit system?
R8-R15
Which flag is used by the program to identify if it is being run in a debugger?
Trap Flag
Which flag will be set when the most significant bit in an operation is set to 1?
Sign Flag
Which Segment register contains the pointer to the code section in memory?
Code Segment
When a program is loaded into Memory, does it have a full view of the system memory? Y or N?
N
Which section of the Memory contains the code?
Code
Which Memory section contains information related to the program’s control flow?
Stack
Follow the instructions in the attached static site and find the flag. What is the flag?
THM{SMASHED_THE_STACK}
Room Answers | TryHackMe x86 Assembly Crash Course
What are the hex codes that denote the assembly operations called?
Opcodes
Which type of operand is denoted by square brackets?
memory operand
In mov eax, ebx, which register is the destination operand?
eax
What instruction performs no action?
nop
Which flag will be set if the result of the operation is zero? (Answer in abbreviation)
ZF
Which flag will be set if the result of the operation is negative? (Answer in abbreviation)
SF
In a subtraction operation, which flag is set if the destination is smaller than the subtracted value?
Carry Flag
Which instruction is used to increase the value of a register
inc
Do the following instructions have the same result? (yea/nay)
xor eax, eax
mov eax, 0
yea
Which flag is set as a result of the test instruction being zero?
Zero flag
Which of the below operations uses subtraction to test two values? 1 or 2?
- cmp eax, ebx
- test eax, ebx
1
Which flag is used to identify whether a jump will be taken or not after a jz or jnz instruction?
Zero Flag
Which instruction is used for performing a function call?
call
Which instruction is used to push all registers to the stack?
pusha
While running the MOV instructions, what is the value of [eax] after running the 4th instruction? (in hex)
0x00000040
What error is displayed after running the 6th instruction from the MOV instruction section?
Memory to memory data movement is not allowed.
Run the instructions from the stack section. What is the value of eax after the 9th instruction? (in hex)
0x00000025
Run the instructions from the stack section. What is the value of edx after the 12th instruction? (in hex)
0x00000010
Run the instructions from the stack section. After POP ecx, what is the value left at the top of the stack? (in hex)
0x00000010
Run the cmp and test instructions. Which flags are triggered after the 3rd instruction?
(Note: Use these abbreviations in alphabetical order with no spaces: CF,PF,SF,ZF)
PF,ZF
Run the test and the cmp instructions. Which flags are triggered after the 11th instruction?
(Note: Use these abbreviations in alphabetical order with no spaces: CF,PF,SF,ZF)
CF,SF
Run the instructions from the lea section. What is the value of eax after running the 9th instruction? (in hex)
0x0000004B
Run the instructions from the lea section. What is the final value found in the ECX register? (in hex)
0x00000045